For changing the color of a column in ALV.
we need to get the column using the interface controller of the ALV used comp
and then set its cell design to the desired color or design .
as shown in the below fig.
here we are getting the column instance of column "STATUS" and set it to some
design using the method set_cell_design
Here is the code
method WDDOINIT .
data lo_cmp_usage type ref to if_wd_component_usage.
lo_cmp_usage = wd_this->wd_cpuse_alv( ).
if lo_cmp_usage->has_active_component( ) is initial.
lo_cmp_usage->create_component( ).
endif.
DATA lo_INTERFACECONTROLLER TYPE REF TO IWCI_SALV_WD_TABLE .
lo_INTERFACECONTROLLER = wd_this->wd_cpifc_alv( ).
DATA lo_value TYPE ref to cl_salv_wd_config_table.
lo_value = lo_interfacecontroller->get_model(
).
*we dont want to display the celldesign as seperate column so remove it form the ALV table
lo_value->IF_SALV_WD_COLUMN_SETTINGS~DELETE_COLUMN( 'CELLDESIGN' ).
* get the column whose cell design you want to change
data col1 type ref to cl_salv_wd_column.
col1 = lo_value->IF_SALV_WD_COLUMN_SETTINGS~GET_COLUMN( 'STATUS' ).
* change the cell design BINDING IT TO THE FIELD 'CELLDESIGN'
col1->SET_CELL_DESIGN( CL_WD_TABLE_COLUMN=>E_CELL_DESIGN-NEGATIVE ). endmethod.
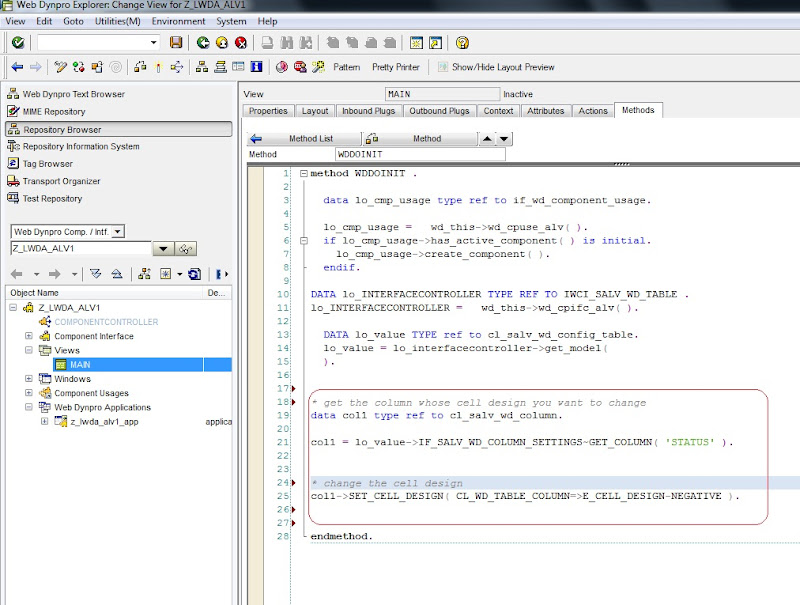
then as we can see the column 'status' is coming in different color.
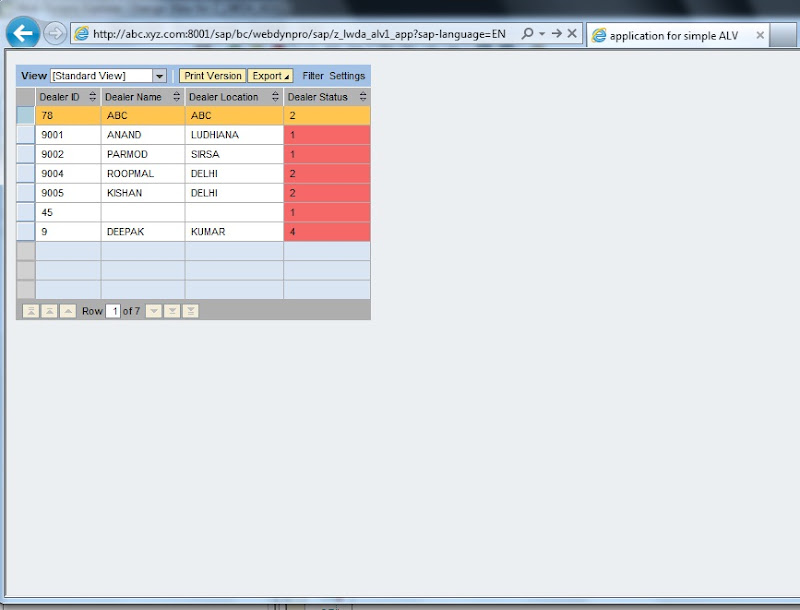
Now we will have a look at one example where we will change the color of cell based on some condition. here in this example we will change the cell color based on the content of the status
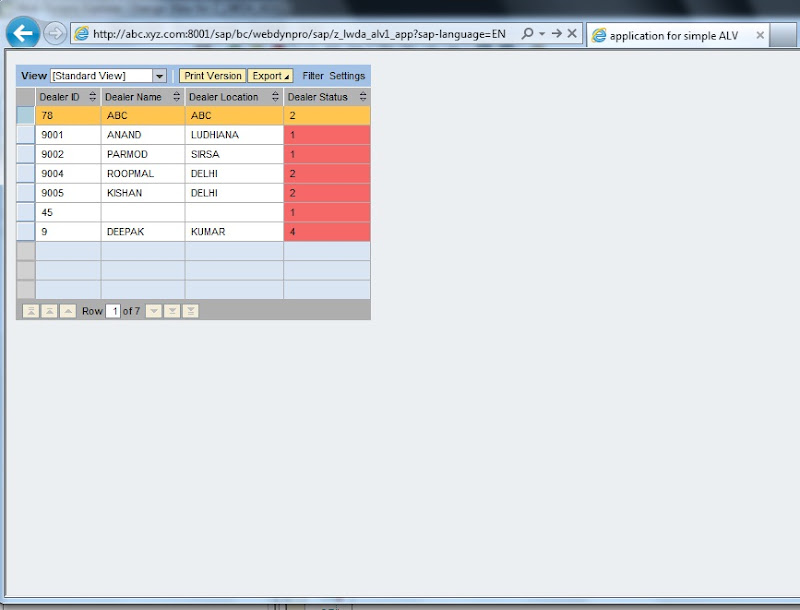
Now we will have a look at one example where we will change the color of cell based on some condition. here in this example we will change the cell color based on the content of the status
column.
For this, follow the steps as listed below.
1. go to the component controller having the node which is acting as source for the ALV table.
there add one more attribute ( name celldesign and type WDUI_TABLE_CELL_DESIGN )
Add the same attribute to view controller context also.
2. then in the wddoinit method. get the column and set its property cell design property to some field name. here we will set it to CellDesign field. then modify the data of the node to fill the
attribute CELLDESIGN according to the content of the status field.
then fill the node with new modified values.
here is the code..
method WDDOINIT .
data lo_cmp_usage type ref to if_wd_component_usage.
lo_cmp_usage = wd_this->wd_cpuse_alv( ).
if lo_cmp_usage->has_active_component( ) is initial.
lo_cmp_usage->create_component( ).
endif.
DATA lo_INTERFACECONTROLLER TYPE REF TO IWCI_SALV_WD_TABLE .
lo_INTERFACECONTROLLER = wd_this->wd_cpifc_alv( ).
DATA lo_value TYPE ref to cl_salv_wd_config_table.
lo_value = lo_interfacecontroller->get_model(
).
*we dont want to display the celldesign as seperate column so remove it form the ALV table
lo_value->IF_SALV_WD_COLUMN_SETTINGS~DELETE_COLUMN( 'CELLDESIGN' ).
* get the column whose cell design you want to change
data col1 type ref to cl_salv_wd_column.
col1 = lo_value->IF_SALV_WD_COLUMN_SETTINGS~GET_COLUMN( 'STATUS' ).
* change the cell design BINDING IT TO THE FIELD 'CELLDESIGN'
col1->SET_CELL_DESIGN_FIELDNAME( 'CELLDESIGN' ).
*now get the node's element collection into one internal table
*and fill cell design according to the required condition
DATA lo_nd_dealer TYPE REF TO if_wd_context_node.
DATA LT_dealer TYPE wd_this->elementS_dealer.
DATA LS_DEALER TYPE WD_THIS->ELEMENT_DEALER.
lo_nd_dealer = wd_context->get_child_node( name = wd_this->wdctx_dealer ).
* get all elements into internal table
lo_nd_dealer->get_static_attributes_TABLE(
IMPORTING
TABLE = LT_dealer ).
* loop at the internal table and modify the records as per the requirement
* here in this case i am giving different colors to the cell according to the content
* status 1 is red , 4 is green and rest is some other color
LOOP AT LT_DEALER INTO LS_DEALER.
IF LS_DEALER-STATUS EQ '0001'.
LS_DEALER-CELLDESIGN = CL_WD_TABLE_COLUMN=>E_CELL_DESIGN-NEGATIVE.
ELSEIF LS_DEALER-STATUS EQ '0004'.
LS_DEALER-CELLDESIGN = CL_WD_TABLE_COLUMN=>E_CELL_DESIGN-POSITIVE.
ELSE.
LS_DEALER-CELLDESIGN = CL_WD_TABLE_COLUMN=>E_CELL_DESIGN-BADVALUE_MEDIUM.
ENDIF.
MODIFY LT_DEALER FROM LS_DEALER.
ENDLOOP.
*fll the node with new element collection from the modified internal table lt_dealer
lo_nd_dealer->BIND_TABLE(
LT_DEALER
).
endmethod.
3. save and activate . you will get the application like this.
Thanks
Gill :)